COMPONENTS :
HC-SR04 ultrasonic sensor
Arduino Uno Board
Jumper Wires
Buzzer
THEORY :
In this module, we are using HC-SR04 ultrasonic sensor which emits and receives the ultrasonic wave through trigger and echo respectively and calculate the time taken by the wave to come back after getting reflected from some object. Then we use the formula DISTANCE=SPEED*TIME, where SPEED = 0.034 and we divide it by 2 as it gives the distance covered by the wave to go back and forth. This gives us the distance of the object from the sensor.
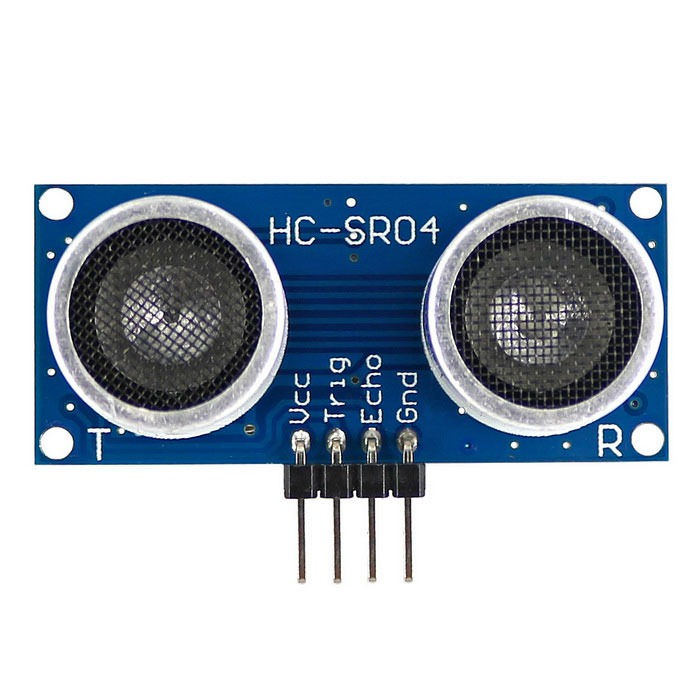
CONNECTION :
1. Connect VCC on the ultrasonic sensor to the 5V pin on the Arduino.
2. Connect the Trig pin on the ultrasonic sensor to pin 2 on the Arduino.
3. Connect the Echo pin on the ultrasonic sensor to pin 3 on the Arduino.
4. Connect the GND on the ultrasonic sensor to GND on the Arduino.
5. Connect the positive pin on the buzzer with pin 10 on the Arduino.
6. Connect the buzzer's negative pin with the GND pin on the Arduino.
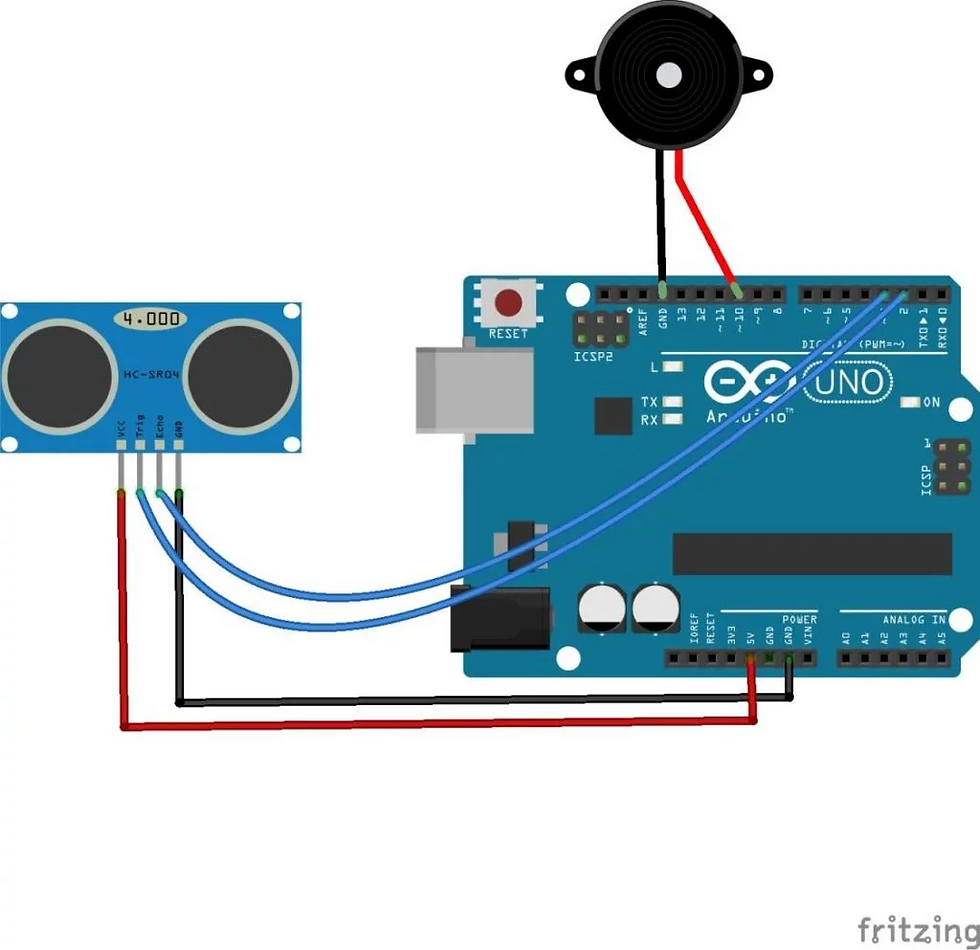
CODE :
int trigger = 2;
int echo = 3;
int buzzer = 10;
int time;
int distance;
void setup ( )
{
Serial.begin (9600);
pinMode (trigger, OUTPUT);
pinMode (echo, INPUT);
pinMode (buzzer, OUTPUT);
}
void loop ( )
{
digitalWrite (trigger, HIGH);
delay(500);
digitalWrite (trigger, LOW);
time = pulseIn (echo, HIGH);
distance = (time * 0.034) / 2;
if (distance <= 10)
{
Serial.println (" Obstacle Detected ");
Serial.print (" Distance= ");
Serial.println (distance);
digitalWrite (buzzer, HIGH);
delay (500);
}
else
{
Serial.println (" No Obstacle Detected ");
Serial.print (" Distance= ");
Serial.println (distance);
digitalWrite (buzzer, LOW);
delay (500);
}
}
// If there is any problem while making this project feel free to ask in the comment.